In this post am going to demonstrate a clinic project to
support requirement which is that patients visit clinic and get registered, after
that, they make an appointment by selecting the populated available doctors,
which in turn lists the upcoming appointments for the selected doctor. By
default the appointment gets a pending status because it needs to be reviewed. After
that, the doctor is going to work out the patient attendance. Under the report we
should have daily and monthly appointments.
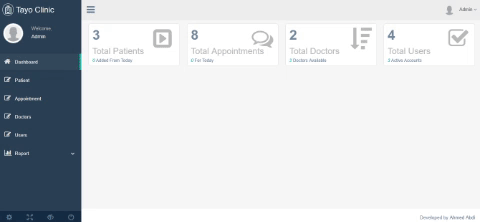
Domain Entities
Now let’s start the domain entities that we had generated
the database using code first migration.The Patient entity has information such as token (auto generated serial
number), name, phone, and Age(derived from birthdate). A patient will have one
or more appointment or attendance, so that we need to add a collection of
appointments and attendances.
public class Patient { public int Id { get; set; } public string Token { get; set; } public string Name { get; set; } public Gender Sex { get; set; } public DateTime BirthDate { get; set; } public string Phone { get; set; } public string Address { get; set; } public byte CityId { get; set; } public City Cities { get; set; } public DateTime DateTime { get; set; } public string Height { get; set; } public string Weight { get; set; } public int Age { get { var now = DateTime.Today; var age = now.Year - BirthDate.Year; if (BirthDate > now.AddYears(-age)) age--; return age; } } public ICollection<Appointment>Appointments { get; set; } public ICollection<Attendance> Attendances { get; set; } public Patient() { Appointments = new Collection<Appointment> (); Attendances = new Collection<Attendance> (); } }
Appointment has date time, details, and status (approved or pending).
public class Appointment { public int Id { get; set; } public DateTime StartDateTime { get; set; } public string Detail { get; set; } public bool Status { get; set; } public int PatientId { get; set; } public Patient Patient { get; set; } public int DoctorId { get; set; } public Doctor Doctor { get; set; } }
Each attendance has information such as diagnosis, therapy and clinic remarks. It has also reference to specific patient.
public class Attendance { public int Id { get; set; } public string ClinicRemarks { get; set; } public string Diagnosis { get; set; } public string SecondDiagnosis { get; set; } public string ThirdDiagnosis { get; set; } public string Therapy { get; set; } public DateTime Date { get; set; } public int PatientId { get; set; } public Patient Patient { get; set; } }
Each doctor has one or more appointments and has also a reference to specific specialization.
public class Doctor { public int Id { get; set; } public string Name { get; set; } public string Phone { get; set; } public bool IsAvailable { get; set; } public string Address { get; set; } public int SpecializationId { get; set; } public Specialization Specialization { get; set; } public string PhysicianId { get; set; } public ApplicationUser Physician { get; set; } public ICollection<Appointment>Appointments { get; set; } public Doctor() { Appointments = new Collection<Appointment> (); } }
Application structure
All persistence ignorant parts are in Core folder; these are domain entities, Dtos, view models, and
interfaces. The Persistence folder
contains entity configurations and the implementation of repository interfaces.
Accounts
There are two users; administrator and doctors.
Admin has full application access including adding new
patient and assign him/her to available doctors.
The doctor is being registered first by an administrator,
and has view that displays the patients assigned to him.
What we used
Server Side
- ASP.NET MVC: Web application development framework.
- Entity Framework: an ORM for accessing data.
- Ninject: an Inversion control container for resolving dependencies.
- Automapper: for mapping domain entities to Dtos.
Front End
- Jquery Datatables.
- Bootbox
- Bootstrap
Design template
I hope this project is useful for beginners. You can get source code from here.
0 comments:
Post a Comment